top of page
Python Program -Zombie Simulation
The Zombie Simulation was a project based on a zombie apocalypse scenario in which there are three groups of people susceptibles, Infected, and Removed.
The Simulation is Based on a model called the SIR Model which is short for Susceptible-Infected-Removed Model.
In the model, the susceptibles are people that are susceptible to being infected, the infected are people who have been zombified and the removed are people that are either cured of infection or have died.
The SIR model also includes equations. Each group in the model has an equation that shows how the group changes over time whether by gaining or losing members. The XYZ Model to the right is a good illustration of how the SIR Model works.

The idea of this model is that X members can become Y members and both X and Y can become Z members. The greek characters determine the rate at which this change happens.
The objective of this project was to create a live python simulation using object-oriented programming to better illustrate the SIR Model.
This simulation is considered live because, upon the creation of the classes and objects of each group, the members become instances that actually move around the map. The snippet to the left shows how the movements work.

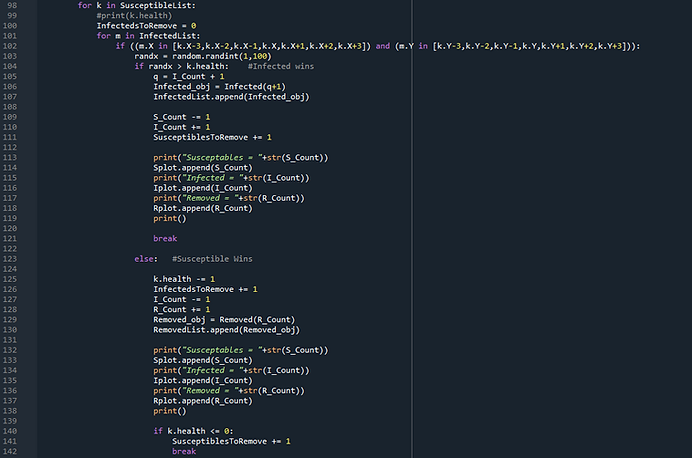
This is a small section of the code to show what happens when a susceptible object encounters an Infected Object.
The bulk of the code is the for loops that show how each group changes over time. When a Susceptible and an Infected encounter each other they fight and there is a chance that a susceptible could become infected or an infected could die and become removed. This is all based on the random amount of health a susceptible member has. So, the expected result of this simulation is that the number of susceptible and infected should fall while the number of removed rise.
Using the matplotlib.pyplot library we plot the results by creating a line graph of how the amount of each member from the groups changes over time.
The results of the simulation are exactly what we expect because just like we can see in the SIR model the number of Removed rises while the number of Susceptables and Infected falls. This Simulation is an applicable description of the SIR model because even though components change the results remain the same.

What the console output shows from running the simulation. It continues online we run out of susceptibles or removed.

C Program - Sudokoboard Validator
The requirement of this project was to create a multithreading application in C that had the functionality of checking a sudoku puzzle and telling the user if the board had been solved or not.
For a sudoku board to be completed, each column, row, and 9x9 subgrid must contain digits from 1-9 in which each digit only appears once for each segment.​

To accomplish the goal I created three threads.
-
One that would check each column of the board.
-
One that would check each row of the board.
-
One that would check each 9x9 subgrid of the board.
Each thread would validate its portion of the board and return if it is correct or not.
If all three treads return valid then the sudoku board is valid otherwise it's not.
The section of the code to the right illustrates the creation of the three threads that validate the board. The link to the entire code can be found at the bottom of the image.

bottom of page